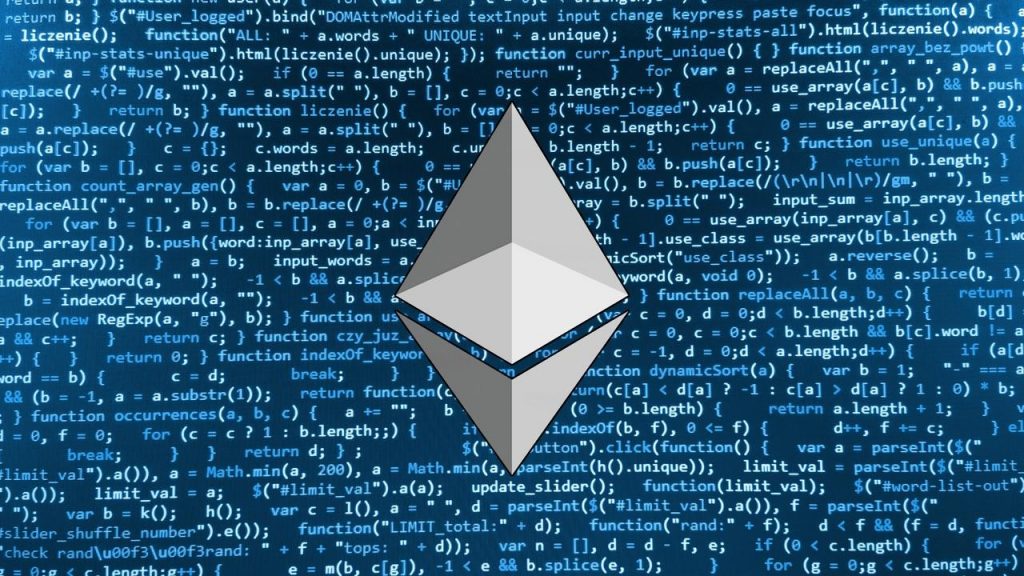
Ethereum is one of the most fascinating and impactful projects in the crypto space. By bringing in the idea of programmable blockchains, Ethereum pretty much ushered in the era of smart contract platforms. Now with Ethereum 2.0 just around the corner, let’s familiarize ourselves with Ethereum code.
Ethereum Code: The Ins and Outs of Ethereum smart contracts
What code is Ethereum written in? How to code Ethereum smart contracts? What are ERC-20 tokens? Let’s answer all these questions in this guide.
What code is Ethereum written in?
Vitalik Buterin, a Russian-Canadian programming prodigy, first released the Ethereum whitepaper in 2013, describing it as a platform that can accommodate decentralized applications (Apps). Ethereum has a long list of co-founders and was coded using– Go, Rust, C#, C++, Java, and Python.
However, when learning about Ethereum code, this is not the main information that most developers are looking for. The truly fascinating aspect of Ethereum code lies in smart contracts.
How to code Ethereum smart contracts?
Finally, let’s get into smart contracts. Smart contracts are self-executing, automated, and programmable agreements between two parties. The core idea is to directly connect two parties to each other without having to go through a third-party or an intermediary. So, how exactly do you code these smart contracts?
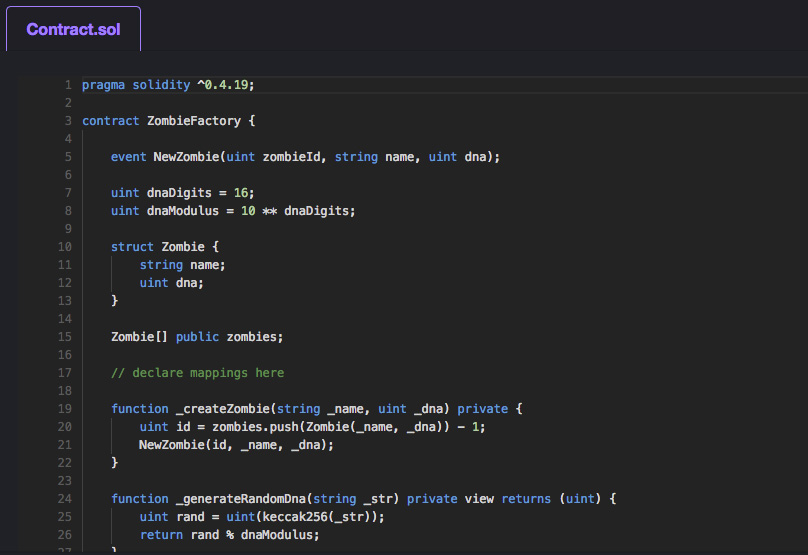
Ethereum code: Solidity
Solidity is a purposefully slimmed down, loosely-typed language with a syntax very similar to JavaScript. Solidity-coded smart contracts can be easily executed in the Ethereum Virtual Machine or the EVM.
Every line of code in these contracts cost a certain amount of computational resource called “gas.” Before the execution, the contract comes with a preset gas limit, which is the maximum amount of gas that the contract will consume for its implementation. After you write your code, you will compile it using the “solc” compiler.
Solidity data types
Data types allow a program to specify and classify all the data they are using within the code. Here are the different data types within Solidity:
- Signed integers or “int” that goes from -128 to 127
- Unsigned Integer “uint” that goes from 0-255 and doesn’t store any negative values
- Boolean data types or “bool” can store only two values “true” or “false.”
- Store an array of bytes that goes from 1-32 characters in the “bytes” data type.
- Store an array of values in the “string” data type.
- You can use “enum” to create customizable data types.
Basic Solidity Program #1: Hello World
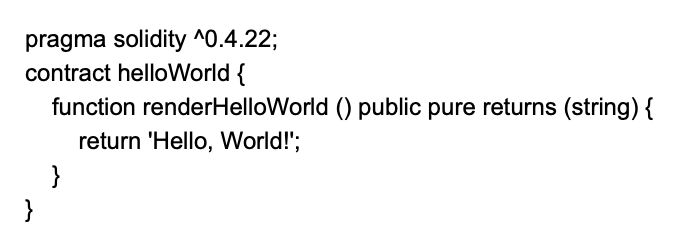
What we have here is the standard “Hello World” program. The moment you call this contract, it’s going to print “Hello World.”
Basic Solidity Program #2: Name and Age
Now, let’s instead take a look at a more complex program.
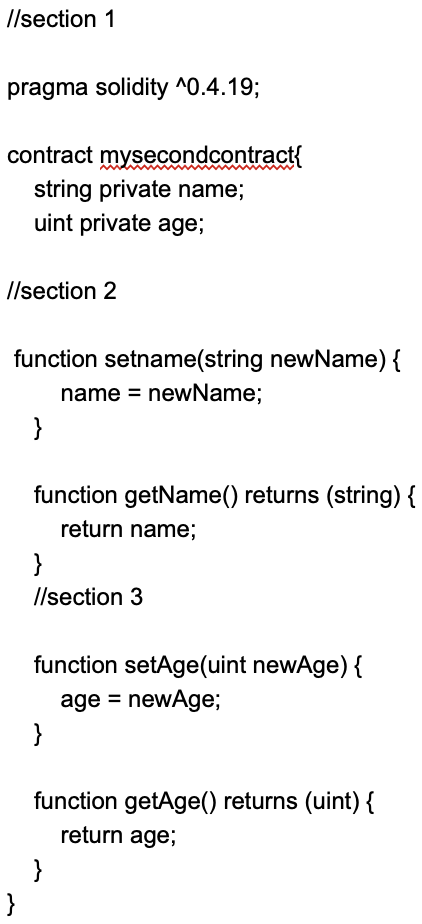
Alright, so now we have a considerably longer program. As you can see, we have broken it down into three sections – Section 1, Section 2, and Section 3.
- Section 1: We have defined the smart contract and its data types. It has one string (name) and one unsigned integer (age).
- Section 2: In this section, we are getting the name from our user.
- Section 3: In this section, we are getting their age.
Once again, it’s a very simple code. However, this is exactly the kind of program you need to master before dealing with more sophisticated smart contracts.
Ethereum code deployment process
The workflow for proper smart contracts deployment looks something like this:
- Setup your Ethereum node. “Geth” is a pretty popular Ethereum client.
- Create and compile your solidity contract.
- Deploy the contract to the network by setting a gas limit
If you want to start coding contracts, the following tools will be very handy.
Ethereum code tool #1: Truffle and Embark
Truffle and Embark are two very useful tools that can help you out immensely in the beginning. Truffle simplifies some of the more mundane and complicated processes for you. Embark, on the other hand, provides a great sandbox platform for you to play around with your dApp.
Ethereum code tool: #2 IDEs
The Remix IDE is a powerful, open-source tool that was developed by the Ethereum Foundation. It is coded in JavaScript and enables you to test out your solidity contracts in-browser.
Remix’s screen looks something like this:
So, what’s going on here? Let’s move from left to right.
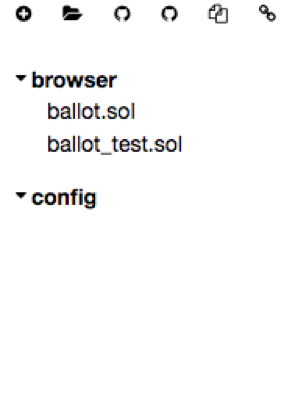
This section has all the smart contracts that you are currently working on. In this case its ballot and ballot_test.
Up next you have this:
This is the editor where you are actually going to code. By default, the Remix UI has the ballot smart contract which you can ignore for now. There is no need to get into this.
Just below this section, you can see this screen:
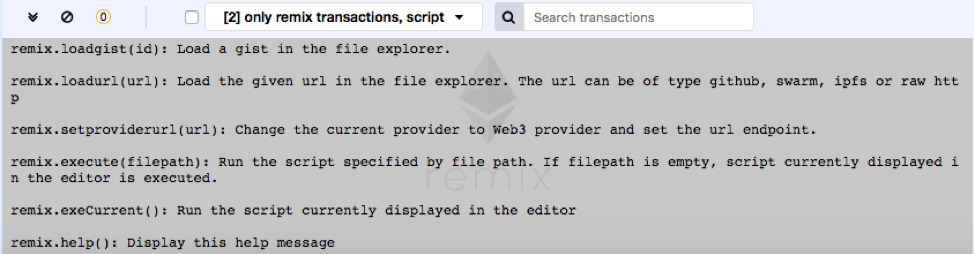
This is the program log section that records all the errors you made during contract execution.
On the right side you have:
The “Run Tab” is where you are going to do most of the work.
There are four fields here that we need to look into:
- Environment: There are three environments where you can execute your smart contracts – JavaScript VM, Injected Web3, and Web3 Provider.
- Account: There are five account addresses that you can use as an owner of this contract. Each address has 100 test ether tokens attached to it.
- Gas Limit: The total gas limit that is assigned for the execution of this smart contract.
- Value: How much Ether tokens you want to provide for your transactions.
Ethereum code alternative: Vyper
Quite like Solidity, Vyper is also a smart contract language that is compatible with the EVM. However, instead of emulating Javascript, Vyper is heavily inspired by the design philosophy of Python. Solidity contracts end with “.sol.” On the other hand, Vyper contracts end with “.vy”.
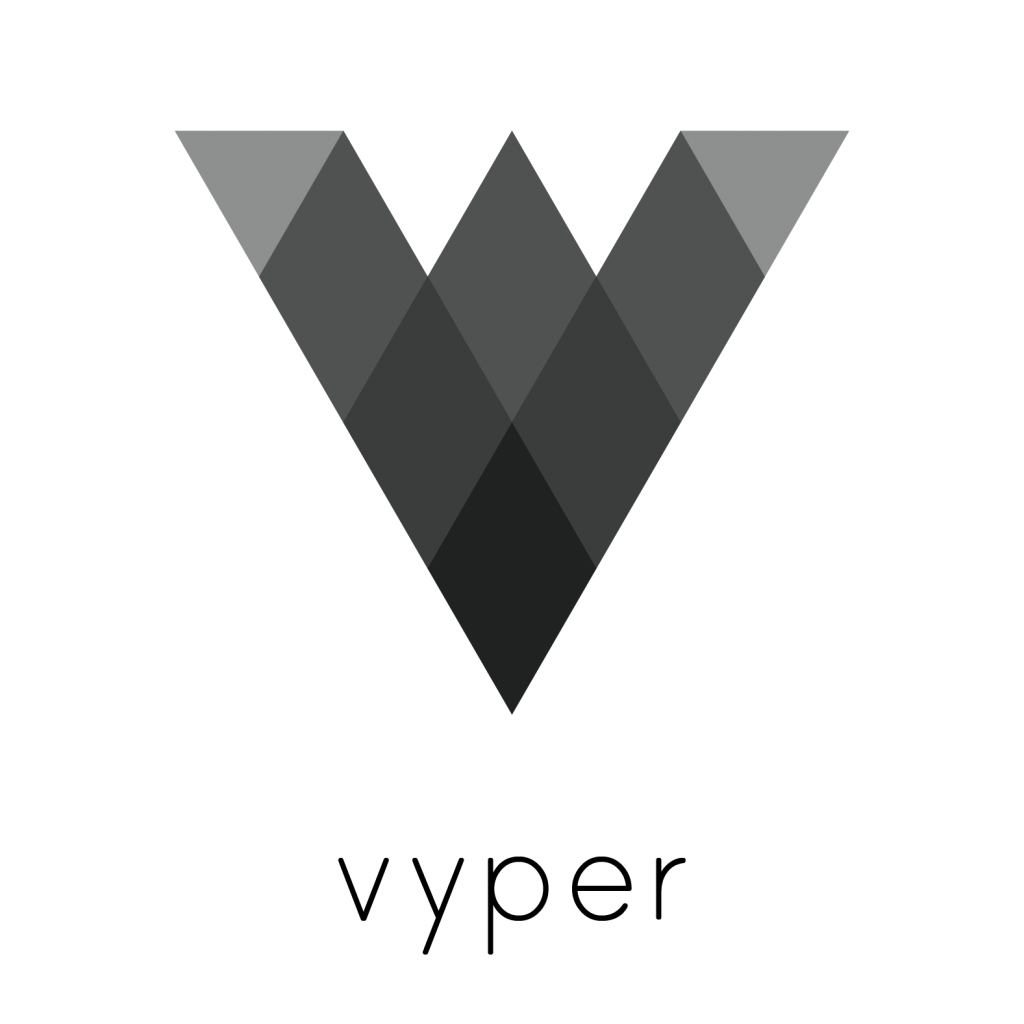
There are two principles that vyper strictly adheres to – Simplicity and Security.
#1 Simplicity
Vyper emphasizes code simplicity above everything else. It does so by doing away with certain traditional constructs such as class inheritance, function overloading, operator overloading, and recursion. These constructs increase complexity and can be vulnerable points during contract execution. Plus, none of them are necessary for Turing-complete contract execution.
#2 Security
Along with simplicity, Vyper emphasizes security. Vyper was never designed to be a solidity substitute. It’s an alternative language that developers can use when security is paramount.
ERC-20 token design
Every single dApp built on top of Ethereum can have its own native token. However, those tokens are ruled by a set of principles. As per Wikipedia, ERC-20 is:
- A list of rules that an Ethereum-based token must integrate.
- A set of guidelines that the developer must follow while creating their contract.
- It is simple to deploy and interoperable with other Ethereum token standards.
Why standardize the design?
So, why have we standardized these token designs? Well, imagine that you live in a country which uses five different kinds of currencies. Certain stores only use currency 1, while the other only uses currency 3, and so forth. Can you imagine how much of a nightmare it will be to live in that country?
You can extend the same logic to Ethereum dApps.
Back in the heyday of ICOs, each and every project was trying to do something innovative and different with their tokens. However, this reduced interoperability drastically. To create a healthy ecosystem on top of Ethereum, the dApps should be able to seamlessly interact with each other.

Fabian Vogelsteller
On November 19, 2015, Fabian Vogelsteller had a solution to this significant problem.
ERC-20: What is it?
As we have mentioned before, ERC-20 is a list of rules and regulations that developers must use to create their tokens. Let’s break down the name itself. “ERC” means Ethereum Request for Comment, while “20” is the number assigned to this request. ERC-20 consists of three optional rules and six mandatory rules.
The six mandatory rules:
- totalSupply
- balanceOf
- transfer
- transferFrom
- approve
- allowance
The three optional rules
- Token Name
- Symbol
- Decimal (up to 18)
ERC-20: Three optional rules
- Token Name: While it isn’t necessary to name your tokens, you should do it to give it some identity.
- OMG, COMP, etc. are all symbols that often get more widely known than the main project itself (OmiseGo and Compound, in this case).
- ERC-20 tokens can be made divisible thanks to the decimal point rule. The decimal point of “3” means that the lowest value that the token is divisible to is 3. The maximum amount of divisibility allowed is 18.
ERC-20: Six mandatory rules
Rule 1: totalSupply
The total supply of the native token in the ecosystem. The code looks like this:
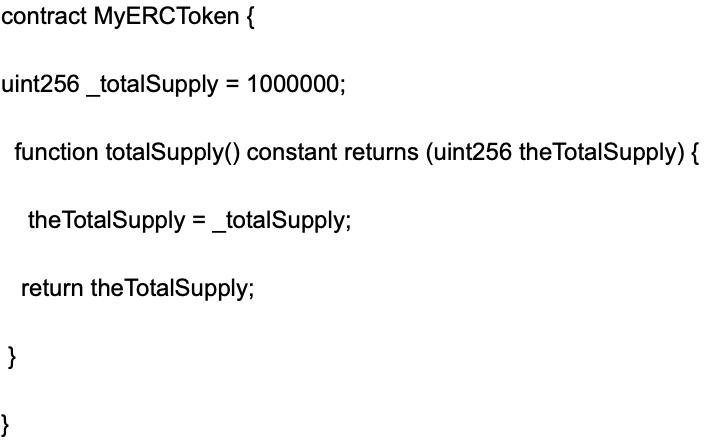
Rule 2: balanceOf
Returns the total number of tokens that the contract owner has in their account. The code looks like this:
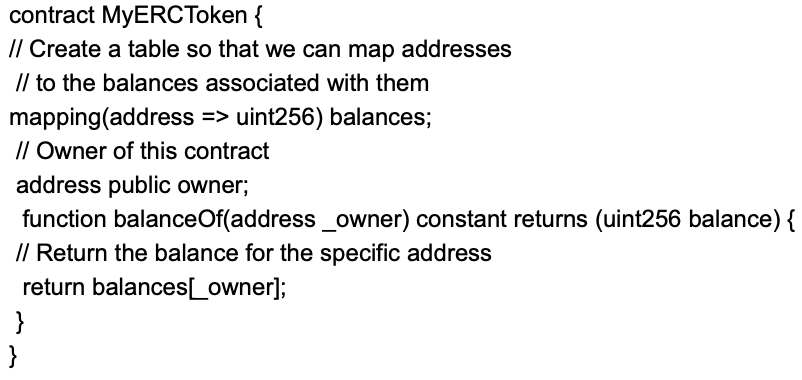
Rule 3: approve()
The approve() function kicks in to allow users to collect tokens from the contract address. Let’s take a look at how this function looks like:
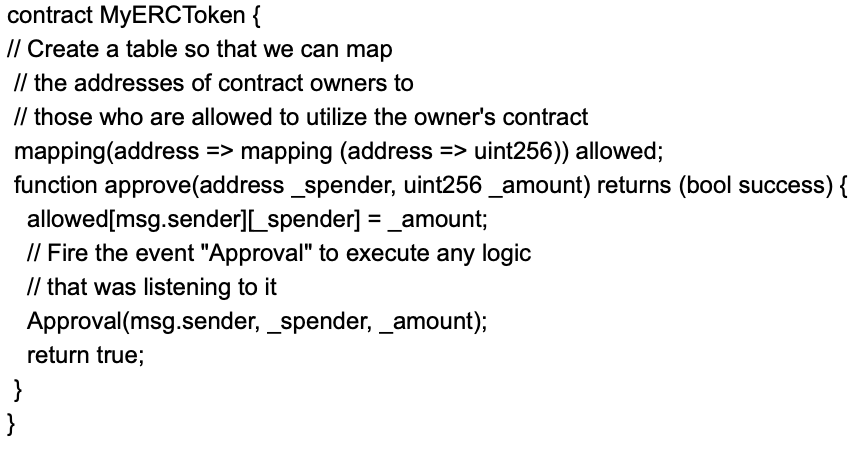
Rule 4: transfer()
Alright, so the contract has checked the balance and approved the transaction. The next step is to transfer these tokens with the transfer() function. The contract owner can send the tokens as a conventional cryptocurrency transaction. This is what the code looks like:
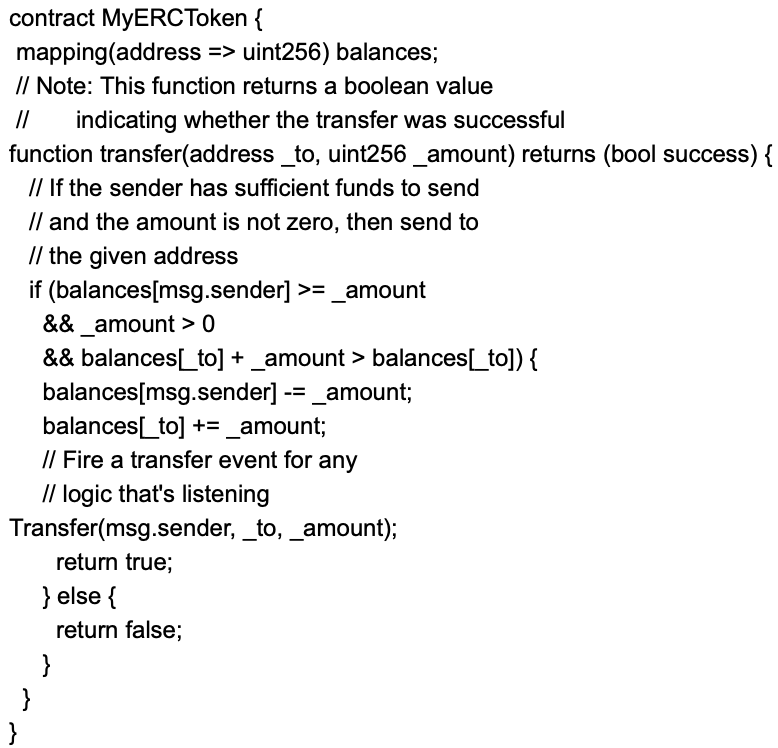
Rule 5: transferFrom()
This function is pretty similar to transfer(), but there is one small difference. Imagine this situation:
You are a restaurant owner and you have a weekly arrangement with your local fishmonger. In this case, instead of paying them all the time like clockwork, you can simply have a system set up between your bank account and theirs. Your account executed a payment routinely and automatically.
As you can imagine, this reduces overhead by a huge amount. This is exactly what we can achieve with transferFrom(). Let’s look at the code:
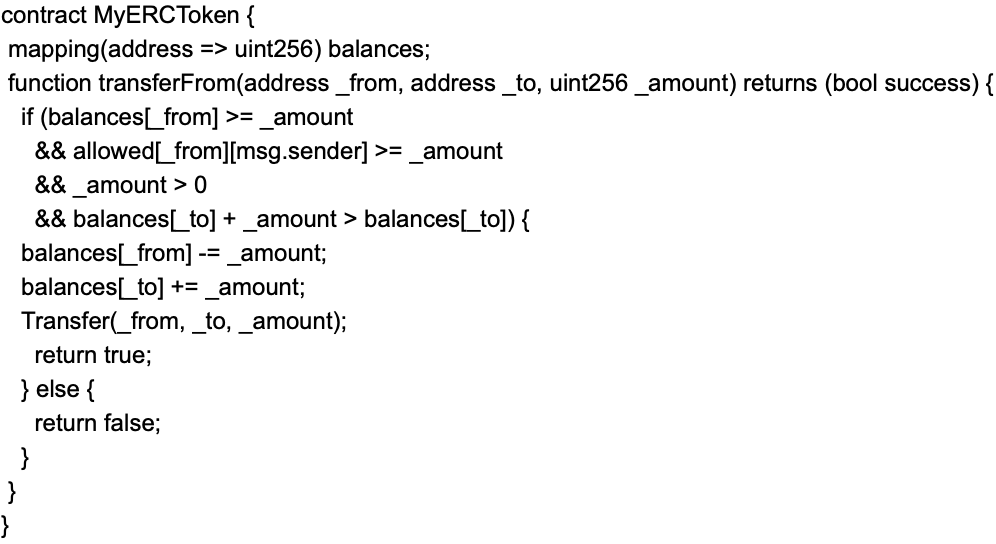
Rule 6: allowance()
When you think about it, what’s the core necessity of a transaction? You can only send a transaction if you have the minimum balance in your account to do it, right? This is why ERC-20 contracts have the allowance() function to do this very check. Now, let’s take a look at the code:

POINT TO NOTE
Golem’s GNT is one of the most well-known tokens out there. However, it lacks the approve() and transferFrom() functions. This is why GNT isn’t considered ERC-20 compliant.
Ethereum code: Conclusion
There you have it! Ethereum is one of the most exciting projects in the world out there due to its programmable and customizable nature. Learning how to code smart contracts and creating ERC-20 tokens can open up a whole new avenue for you. We tried to give you a strong base in the article by showing you:
- How to code Ethereum smart contracts?
- What code is Ethereum written in?
- What is ERC-20?
Do you want to take a deeper dive?
If yes, then do check out our world-famous blockchain education courses at Ivan on Tech Academy. Experts from all around the world have carefully curated these courses. So, what are you waiting for? Come on over and sign up!